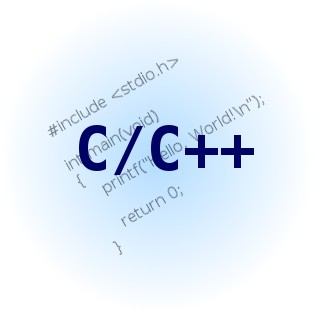
IMPLEMENTATION OF LRU PAGE REPLACEMENT ALGORITHM
AIM:
To write a c program to implement
LRU page replacement algorithm
ALGORITHM :
1. Start the process
2. Declare the size
3. Get the number of pages to be inserted
4. Get the value
5. Declare counter and stack
6. Select the least recently used page by counter value
7. Stack them according the selection.
8. Display the values
9. Stop the process
PROGRAM:
#include<stdio.h>
main()
{
int q[20],p[50],c=0,c1,d,f,i,j,k=0,n,r,t,b[20],c2[20];
printf("Enter no of
pages:");
scanf("%d",&n);
printf("Enter the reference
string:");
for(i=0;i<n;i++)
scanf("%d",&p[i]);
printf("Enter no of
frames:");
scanf("%d",&f);
q[k]=p[k];
printf("\n\t%d\n",q[k]);
c++;
k++;
for(i=1;i<n;i++)
{
c1=0;
for(j=0;j<f;j++)
{
if(p[i]!=q[j])
c1++;
}
if(c1==f)
{
c++;
if(k<f)
{
q[k]=p[i];
k++;
for(j=0;j<k;j++)
printf("\t%d",q[j]);
printf("\n");
}
else
{
for(r=0;r<f;r++)
{
c2[r]=0;
for(j=i-1;j<n;j--)
{
if(q[r]!=p[j])
c2[r]++;
else
break;
}
}
for(r=0;r<f;r++)
b[r]=c2[r];
for(r=0;r<f;r++)
{
for(j=r;j<f;j++)
{
if(b[r]<b[j])
{
t=b[r];
b[r]=b[j];
b[j]=t;
}
}
}
for(r=0;r<f;r++)
{
if(c2[r]==b[0])
q[r]=p[i];
printf("\t%d",q[r]);
}
printf("\n");
}
}
}
printf("\nThe no of page faults is %d",c);
}
OUTPUT:
Enter no of pages:10
Enter the reference string:7 5 9 4
3 7 9 6 2 1
Enter no of frames:3
7
7
5
7
5 9
4
5 9
4 3 9
4
3 7
9
3 7
9
6 7
9
6 2
1
6 2
The no of page faults is 10
16 comments:
thank u!
great
thank u for helping ..
Thank u so much! Thanks from Kazakhstan
thank u.. very much
thank you.....
one question please
what is r , f, c...
hi, i just have one question please
what is the variable r, f, c, c2??
goooooooood
thank u
does it run in linux??
from norway, thanks!
Thank YoU..!!
Thank u for such a nice code......
thank u ... can u say what is c,c2,k,r
Your algorithm is a bit off at some parts when I tried with different inputs.
Post a Comment