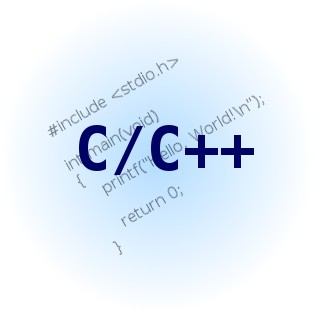
IMPLEMENTATION
OF SHORTEST JOB FIST SCHEDULING ALGORITHM
AIM
To implement the c program for shortest
job first scheduling algorithm
ALGORITHM
1. Start the process
2. Declare the array size
3. Get the number of elements to be inserted
4. Select the process which have shortest burst will execute
first
5. If two process have same burst length then FCFS
scheduling algorithm used
6. Make the average waiting the length of next process
7. Start with the
first process from it’s selection as above and
let other process to be in
queue
6. Calculate the total number of burst time
7. Display the values
8. Stop the process
PROGRAM:
#include<stdio.h>
int main()
{
int n,j,temp,temp1,temp2,pr[10],b[10],t[10],w[10],p[10],i;
float att=0,awt=0;
for(i=0;i<10;i++)
{
b[i]=0;w[i]=0;
}
printf("enter
the number of process");
scanf("%d",&n);
printf("enter
the burst times");
for(i=0;i<n;i++)
{
scanf("%d",&b[i]);
p[i]=i;
}
for(i=0;i<n;i++)
{
for(j=i;j<n;j++)
{
if(b[i]>b[j])
{
temp=b[i];
temp1=p[i];
b[i]=b[j];
p[i]=p[j];
b[j]=temp;
p[j]=temp1;
}
}
}
w[0]=0;
for(i=0;i<n;i++)
w[i+1]=w[i]+b[i];
for(i=0;i<n;i++)
{
t[i]=w[i]+b[i];
awt=awt+w[i];
att=att+t[i];
}
awt=awt/n;
att=att/n;
printf("\n\t
process \t waiting time \t turn around time \n");
for(i=0;i<n;i++)
printf("\t p[%d] \t %d \t\t %d
\n",p[i],w[i],t[i]);
printf("the
average waitingtimeis %f\n",awt);
printf("the
average turn around time is %f\n",att);
return
1;
}
OUTPUT:
enter the number of process 5
enter the burst times
2
4 5 6 8
process waiting time turn around time
p[0]
0 2
p[1]
2 6
p[2]
6 11
p[3]
11 17
p[4]
17 25
the average waitingtime is 7.200000
the average turn around time is
12.200000
7 comments:
can yu pls post the program for the processes which has same burst time...
can you pls post the prog for the sjf in which the processes have the same burst time...
do you plz give separate program for pre emptive and non pre emptive sjf.....
Earlist deadline first
#include
#include
#include
void main()
{
char p[10][5],temp[5];
int tot=0,wt[10],pt[10],i,j,n,temp1;
float avg=0;
clrscr();
printf("enter no of processes:");
scanf("%d",&n);
for(i=0;ipt[j])
{
temp1=pt[i];
pt[i]=pt[j];
pt[j]=temp1;
strcpy(temp,p[i]);
strcpy(p[i],p[j]);
strcpy(p[j],temp);
}
}
}
wt[0]=0;
for(i=1;i<n;i++)
{
wt[i]=wt[i-1]+et[i-1];
tot=tot+wt[i];
}
avg=(float)tot/n;
printf("p_name\t P_time\t w_time\n");
for(i=0;i<n;i++)
printf("%s\t%d\t%d\n",p[i],et[i],wt[i]);
printf("total waiting time=%d\n avg waiting time=%f",tot,avg);
getch();
}
OUTPUT:
enter no of processes: 5
Enter process1 name: aaa
enter process time: 4
enter process2 name: bbb
enter process time: 3
enter process3 name: ccc
enter process time: 2
enter process4 name: ddd
enter process time: 5
enter process5 name: eee
enter process time: 1
p_name P_time w_time
eee 1 0
ccc 2 1
bbb 3 3
aaa 4 6
ddd 5 10
total waiting time=20
avg waiting time=4.00
please send program for shortest job first preemptive with arrival time
here is your solution
#include
struct proc
{
int pid;
int at,bt,wt,tat,rbt;
int flag,flag1;
};
struct proc p1[10];
int i,j,k,n,no,m;
float atat=0.0,awt=0.0;
int tbt=0;
int minimum1();
int main()
{
int minv,locv,mins,locs;
printf("\nenter the number of processes:");
scanf("%d",&n);
printf("\nenter the proc information:");
printf("\nprocess id arrival time burst time");
for(i=0;i0&&p1[j].at<=i)
{
p1[j].flag=1;
}
}
no=minimum1();
printf("%d p[%d]",i,p1[no].pid);
p1[no].rbt=p1[no].rbt-1;
for(k=0;k0&&p1[k].at<=i&&k!=no)
{
p1[k].wt++;
}
}
}
printf("%d",tbt+minv);
for(i=0;i0&&p1[z].at<=i&&p1[z].rbt<mini)
{
mini=p1[z].rbt;
loc=z;
}
}
return loc;
}
in the sorting of shortest job,,,can u try this ex program and practical
the burst time for 8 process were 20,4,3,3,2,4,1,2 respectively
Post a Comment