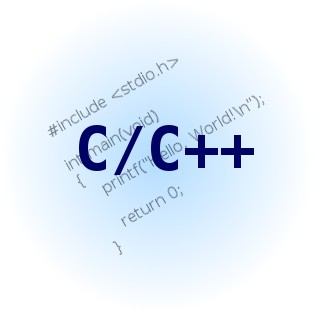
To perform
2D transformations such as translation, scaling, and rotation
on 2D object
ALGORITHM:
1.
Start
2.
Initialize the graphics mode.
3.
Construct a 2D object (use Drawpoly()) e.g. (x,y)
4.
A) Translation
a.
Get the translation value tx, ty
b.
Move the 2d object with tx, ty (x’=x+tx,y’=y+ty)
c.
Plot (x’,y’)
5.
B)
Scaling
a.
Get the scaling value Sx,Sy
b.
Resize the object with Sx,Sy (x’=x*Sx,y’=y*Sy)
c.
Plot (x’,y’)
6.
C) Rotation
a.
Get the Rotation angle
b.
Rotate the object by the angle ф
x’=x cos ф - y sin ф
y’=x sin ф - y cosф
c.
Plot (x’,y’)
PROGRAM:
#include <graphics.h>
#include <stdlib.h>
#include <stdio.h>
#include <conio.h>
#include<math.h>
void main()
{
int gm;
int
gd=DETECT;
int
x1,x2,x3,y1,y2,y3,nx1,nx2,nx3,ny1,ny2,ny3,c;
int
sx,sy,xt,yt,r;
float t;
initgraph(&gd,&gm,"c:\tc\bg:");
printf("\t
Program for basic transactions");
printf("\n\t
Enter the points of triangle");
setcolor(1);
scanf("%d%d%d%d%d%d",&x1,&y1,&x2,&y2,&x3,&y3);
line(x1,y1,x2,y2);
line(x2,y2,x3,y3);
line(x3,y3,x1,y1);
getch();
printf("\n
1.Transaction\n 2.Rotation\n 3.Scalling\n 4.exit");
printf("Enter
your choice:");
scanf("%d",&c);
switch(c)
{
case
1:
printf("\n
Enter the translation factor");
scanf("%d%d",&xt,&yt);
nx1=x1+xt;
ny1=y1+yt;
nx2=x2+xt;
ny2=y2+yt;
nx3=x3+xt;
ny3=y3+yt;
line(nx1,ny1,nx2,ny2);
line(nx2,ny2,nx3,ny3);
line(nx3,ny3,nx1,ny1);
getch();
case
2:
printf("\n
Enter the angle of rotation");
scanf("%d",&r);
t=3.14*r/180;
nx1=abs(x1*cos(t)-y1*sin(t));
ny1=abs(x1*sin(t)+y1*cos(t));
nx2=abs(x2*cos(t)-y2*sin(t));
ny2=abs(x2*sin(t)+y2*cos(t));
nx3=abs(x3*cos(t)-y3*sin(t));
ny3=abs(x3*sin(t)+y3*cos(t));
line(nx1,ny1,nx2,ny2);
line(nx2,ny2,nx3,ny3);
line(nx3,ny3,nx1,ny1);
getch();
case
3:
printf("\n
Enter the scalling factor");
scanf("%d%d",&sx,&sy);
nx1=x1*sx;
ny1=y2*sy;
nx2=x2*sx;
ny2=y2*sy;
nx3=x3*sx;
ny3=y3*sy;
line(nx1,ny1,nx2,ny2);
line(nx2,ny2,nx3,ny3);
line(nx3,ny3,nx1,ny1);
getch();
case
4:
break;
default:
printf("Enter
the correct choice");
}
closegraph();
}
6 comments:
is the rotation with respect to origin?
by default it should be about origin.
it should be about origin by default, if not specifically defined
yes..it is the rotation with respect to origin.
Can u give me the points for triangle
in algo it should be
x’=x cos ф - y sin ф
y’=x sin ф + y cosф
Post a Comment